本文通过 html、css、jquery 以及 math.js 实现了一个简单的在线网页计算器,代码非常简单,旨在初学者通过实际项目来掌握基础知识。
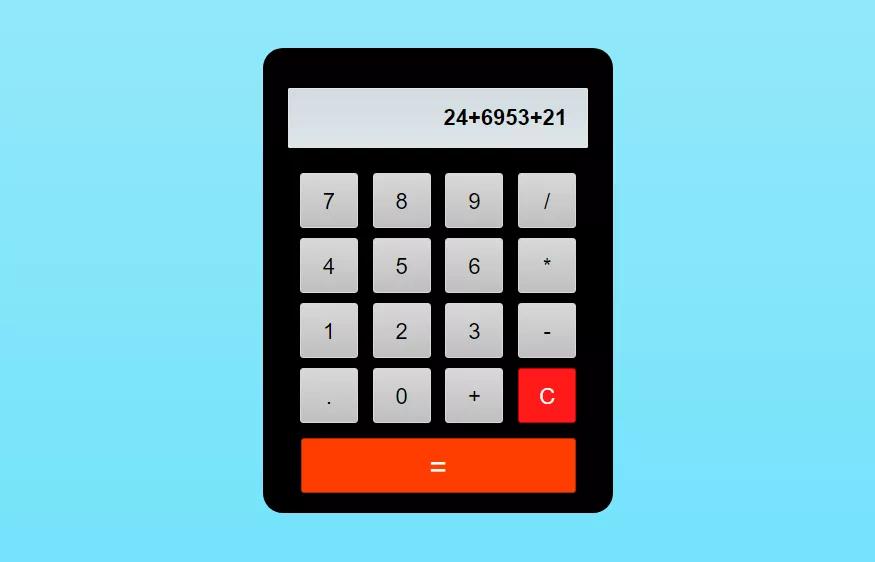
首先,您需要创建两个文件,一个是 html 文件,另一个是 css 文件。创建这些文件后,只需将文中代码粘贴到您的文件中即可。
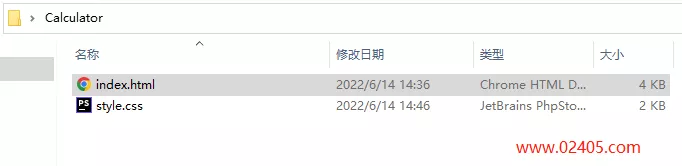
首先,创建一个名为 index.html 的 html 文件,并将下面的代码粘贴到您的 html 文件中。请记住,您必须创建一个扩展名为 .html 的文件。另外一点需要注意的是不要使用 Windows 系统默认的记事本工具。
<!DOCTYPE html>
<!-- Created By www.02405.com -->
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>在线网页计算器</title>
<link rel="stylesheet" href="style.css">
<!--引入 jquery 文件、使用简洁的api来执行js-->
<script src="https://unpkg.com/jquery@3.6.0/dist/jquery.js" type="application/javascript"></script>
<!--引入 math.js 文件、用来进行高精度的数学运算-->
<script src="https://unpkg.com/mathjs/lib/browser/math.js" type="application/javascript"></script>
</head>
<body>
<div class="center">
<form name="forms">
<input type="text" id="display" name="display" disabled>
<div class="buttons">
<input type="button" id="seven" value="7">
<input type="button" id="eight" value="8">
<input type="button" id="nine" value="9">
<input type="button" id="divide" value="/"><br>
<input type="button" id="four" value="4">
<input type="button" id="five" value="5">
<input type="button" id="six" value="6">
<input type="button" id="multi" value="*"><br>
<input type="button" id="one" value="1">
<input type="button" id="two" value="2">
<input type="button" id="three" value="3">
<input type="button" id="subs" value="-"><br>
<input type="button" id="dot" value=".">
<input type="button" id="zero" value="0">
<input type="button" id="add" value="+">
<input type="button" id="clear" value="C"><br>
<input type="button" id="equal" value="=">
</div>
</form>
</div>
<script>
$(document).ready(function(){
$('#one').click(function(){
document.forms.display.value += 1;
});
$('#two').click(function(){
document.forms.display.value += 2;
});
$('#three').click(function(){
document.forms.display.value += 3;
});
$('#four').click(function(){
document.forms.display.value += 4;
});
$('#five').click(function(){
document.forms.display.value += 5;
});
$('#six').click(function(){
document.forms.display.value += 6;
});
$('#seven').click(function(){
document.forms.display.value += 7;
});
$('#eight').click(function(){
document.forms.display.value += 8;
});
$('#nine').click(function(){
document.forms.display.value += 9;
});
$('#zero').click(function(){
document.forms.display.value += 0;
});
$('#add').click(function(){
document.forms.display.value += '+';
});
$('#subs').click(function(){
document.forms.display.value += '-';
});
$('#multi').click(function(){
document.forms.display.value += '*';
});
$('#divide').click(function(){
document.forms.display.value += '/';
});
$('#dot').click(function(){
document.forms.display.value += '.';
});
$('#equal').click(function(){
if (display.value == "") {
alert("请输入要计算的数字!");
}else{
try{
document.forms.display.value = math.format(math.evaluate(document.forms.display.value), {precision: 14});
}catch(e){
alert("输入错误,无法完成计算!");
}
}
});
$('#clear').click(function(){
document.forms.display.value = "";
});
})
</script>
</body>
</html>
其次,在上面的 index.html 文件所在文件夹中创建一个名为 style.css 的 css 文件,并将给定的代码粘贴到您的 css 文件中。请记住,您必须创建一个扩展名为 .css 的文件,同样不要使用 Windows 系统自带的记事本工具。
*{
margin: 0;
padding: 0;
outline: none;
box-sizing: border-box;
}
body{
font-family: montserrat;
display: flex;
text-align: center;
justify-content: center;
align-items: center;
min-height: 100vh;
background: linear-gradient(#9cebfc,#6ae1fb);
}
.center{
/* display: none; */
width: 350px;
background: black;
border-radius: 20px;
}
input[type="text"]{
height: 60px;
width: 300px;
margin-top: 40px;
border-radius: 1px;
border: 1px solid #e1e7ea;
color: black;
font-size: 22px;
font-weight: bold;
text-align: right;
padding-right: 20px;
background: linear-gradient(#d1dce0,#dfe6e9);
}
form .buttons{
width: 300px;
margin: 10px 25px 0 25px;
padding: 10px 0;
}
input[type="button"]{
width: 58px;
height: 55px;
margin: 5px;
font-size: 22px;
line-height: 55px;
border-radius: 3px;
border: 1px solid #d9d9d9;
background: linear-gradient(#d9d9d9, #bfbfbf);
}
input[type="button"]:hover{
transition: .5s;
background: linear-gradient(#bfbfbf, #d9d9d9);
}
input#clear{
background: #ff1a1a;
border: 1px solid #cc0000;
color: white;
}
input#equal{
width: 275px;
margin: 10px 0 10px 0;
font-size: 30px;
color: white;
background: #ff3d00;
border: 1px solid #b32a00;
}
就这么简单,一个在线网页计算器就完成了,接下来右键点击 index.html 文件选择在浏览器中打开就可以运行啦。
链接: https://pan.baidu.com/s/174gVJUGG13G6ssowv9uKHQ?pwd=8tg2 提取码: 8tg2
在线网页计算器源代码下载,由www.02405.com制作分享。
如果你的网页计算器无法正确运行,请发表评论或从联系页面与我们联系。
(adsbygoogle = window.adsbygoogle || []).push({});
来源:https://www.02405.com/archives/5862